Due to Moralis’ Python SDK, integrating blockchain performance into Python tasks is an easy course of! Wish to learn the way to make the most of the SDK so as to add this performance? Comply with alongside as this Web3 py tutorial explores the whole course of from begin to end! Briefly, with the assistance of Moralis and the SDK, you may combine Web3 into Python in two steps:
- Set up the Python SDK from Moralis by working the next command in your terminal:
pip set up moralis
- Make a Moralis API name. Within the code snippet beneath, you can see an instance of what the code may appear like for fetching the native steadiness of a pockets:
from moralis import evm_api api_key = "YOUR_API_KEY" params = { "tackle": "0xd8da6bf26964af9d7eed9e03e53415d37aa96045", "chain": "eth", } outcome = evm_api.steadiness.get_native_balance( api_key=api_key, params=params, ) print(outcome)
Take a look at Moralis’ official Web3 Python SDK documentation for added details about the event package! Furthermore, by signing up with Moralis, you can begin using the code instantly. Nonetheless, should you want additional steerage on using the above code snippet, full this Web3 py tutorial!

Overview
On this tutorial, you’ll learn to combine blockchain performance into your Python tasks. Particularly, the tutorial will train you the right way to create a simple software that includes two central parts: a Python Flask backend app and a frontend React software. Nonetheless, the core focus is directed towards the backend, as that is the place you’ll study the basic rules of mixing Web3 and Python!
Furthermore, due to Moralis and the Python SDK, you may create this app in solely three simple steps:
- Create the Python Flask Software
- Begin the App
- Set Up the React Software
If this sounds attention-grabbing, stick with us by way of this Web3 py tutorial!
Now, the Python SDK is just one of Moralis’ glorious improvement instruments, and in case you are critical about entering into blockchain improvement, we suggest you discover further examples. For example, try the Streams API – one in every of Moralis’ many Web3 APIs. With this software, you may simply stream on-chain knowledge into the backend of your tasks through Moralis webhooks!
Nonetheless, should you plan on following alongside throughout this Web3 py tutorial, be part of Moralis by creating an account, as you want an account to finish the journey herein!
Exploring Web3 Py – What’s it?
Python is a general-purpose, object-oriented programming language that includes an abundance of various use instances. The overall-purpose attribute of Python makes the language versatile. Meaning builders can use it to create every little thing from machine studying tasks to extra simple internet purposes. As well as, Python has an easy-to-learn syntax, making this a best choice for rising builders as that is a straightforward language to undertake.
The earlier paragraph briefly summarizes what Python entails; nevertheless, what does it imply within the context of Web3? First, there are lots of similarities between Web2 and Web3 Python improvement, and the language doesn’t change all that a lot. However, should you come straight from conventional Web2 improvement, there are a number of minor variations it’s best to take into account.
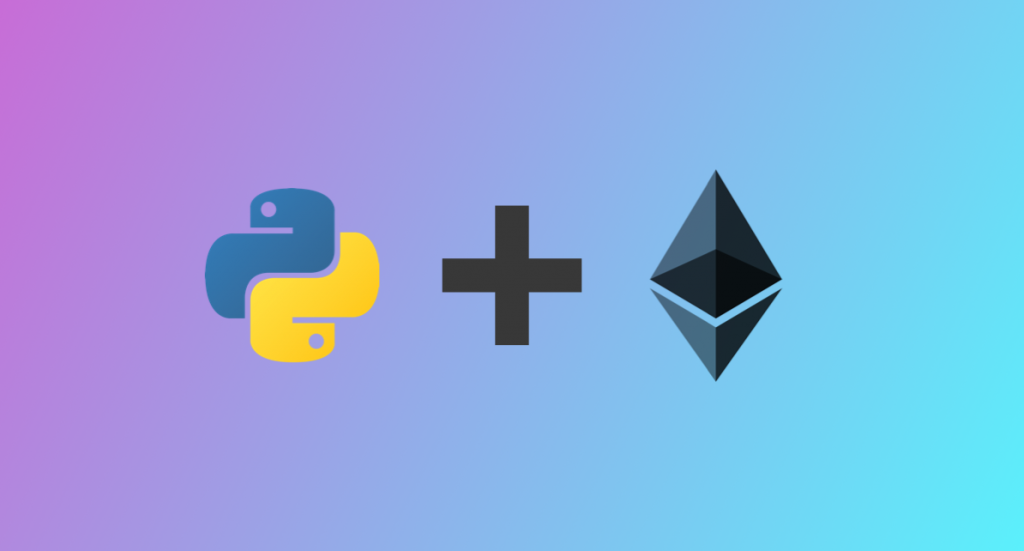
First up, you may need to turn out to be acquainted with the ”Web3.py” library. It is a Web3 Python library enabling you to work together extra seamlessly with the Ethereum community. Moreover, it facilitates a extra accessible developer course of, permitting you to make use of Python for Ethereum improvement.
Aside from libraries, there are further Web3 improvement instruments and platforms you need to try in case you are entering into Web3 py. For example, you need to reap the benefits of Moralis, an industry-leading Web3 infrastructure supplier. With Moralis, you achieve entry to Web3 APIs, SDKs, and rather more, offering a extra simple developer expertise. Amongst Moralis’ options, you can see the Python SDK. With this improvement package, you may simply combine Web3 performance into any of your Python purposes. If you wish to study extra about this superb improvement software, be part of us within the following sections of this Python and Web3 tutorial!
Software Demo – Web3 Py Tutorial Outcomes
Throughout this Web3 py tutorial, you’ll learn to create an software permitting customers to register with MetaMask. Nonetheless, earlier than breaking down this tutorial, we’ll present a fast software demo. In doing so, you’ll achieve a extra profound understanding of what you may be working towards, making it simpler to acknowledge what the underlying code ultimately does. So, with no additional ado, here’s a print display screen of the app’s touchdown web page:
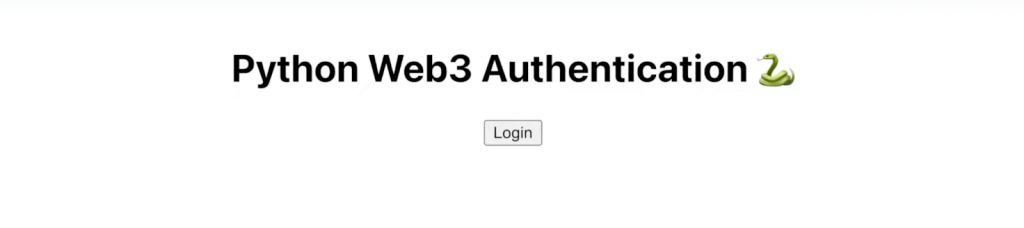
As you may see from the picture, the app’s consumer interface (UI) options two core components: a heading and a button with a ”Login” label. By clicking on this button, it autonomously triggers your MetaMask, prompting you to attach your pockets. As quickly as you join your pockets, the app sends a request to the Python backend, asking Moralis’ API to create a brand new Web3 login problem.
Subsequent up, the app sends one other request liable for validating the signature. In the event that they match, a brand new consumer ID is generated after which displayed on the UI:
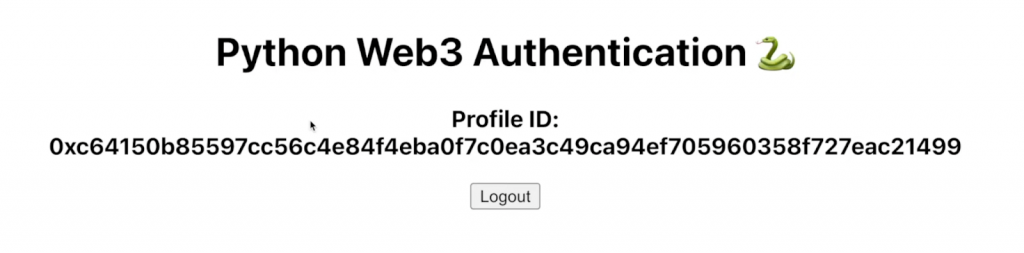
Once you efficiently authenticate, the app makes use of the ID to generate a brand new consumer and provides it to Moralis. Consequently, you may log in to the Moralis admin panel and look at all customers which have signed in below the ”Customers” tab:
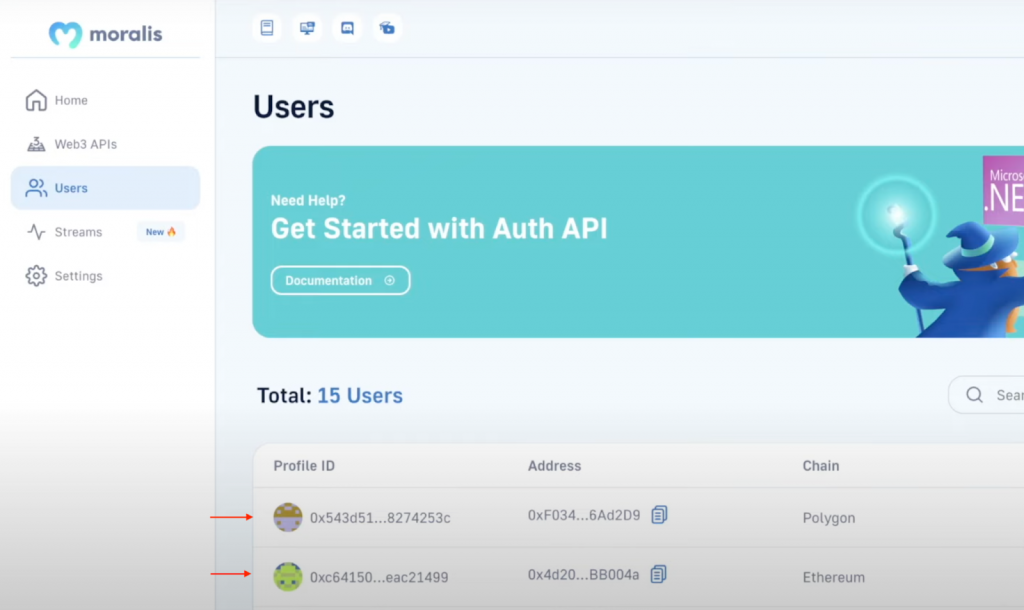
Now that’s it for this temporary demo! Allow us to now soar straight into the Web3 py tutorial to point out you the right way to create this software!
Web3 Py Tutorial
Now that you’ve got familiarized your self with what you’ll work in the direction of, the next sections will train you the right way to create the applying from the earlier part utilizing Moralis and the Python SDK. As talked about earlier, this app consists of two core parts: a Python Flask backend software and a React frontend app.
The central a part of this Web3 py tutorial is the Python Flask app. This app takes care of the logic for dealing with a Web3 authentication circulation, permitting customers to register with their MetaMask wallets. That is additionally the place most of our focus is directed all through the tutorial. Nonetheless, to check out the backend, additionally, you will learn to arrange the frontend app. From it, we’ll name the endpoints and take a look at the Web3 authentication mechanism.
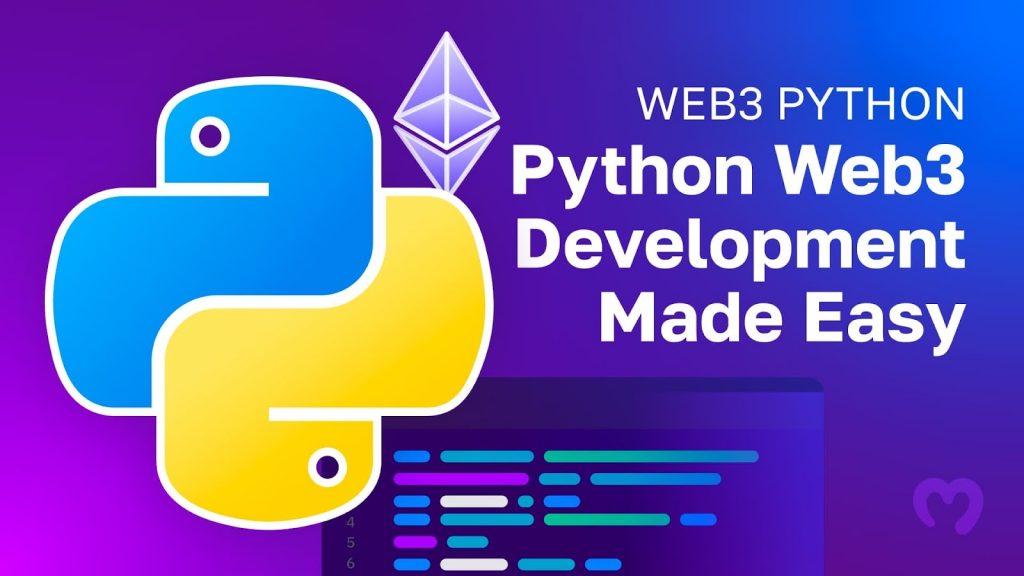
Though we outlined the steps earlier on the right way to full this mission, here’s a temporary reminder of them:
- Create the Python Flask Software
- Begin the App
- Set Up the React Software
By finishing the three steps of this Web3 py tutorial, you’ll learn to arrange a Web3 Python backend software for dealing with Web3 authentication flows! Nonetheless, with no additional ado, allow us to soar straight into step one and carefully study the right way to create the Python Flask software!
Step 1: Create the Python Flask Software
Earlier than leaping into the Python Flask software code, this preliminary a part of the Web3 py tutorial illustrates the right way to arrange the barebones state of the mission. As such, to start with, open your built-in improvement surroundings (IDE) and create a Python folder.
Moreover, all through this Web3 py tutorial, we’ll make the most of Visible Studio Code (VSC). As such, should you choose utilizing one other IDE, keep in mind that the method may differ generally. Nonetheless, with an empty mission folder at your disposal, go forward and launch a brand new terminal. If you happen to, like us, are utilizing VSC, click on on the ”Terminal” tab on the high, adopted by ”New Terminal”:
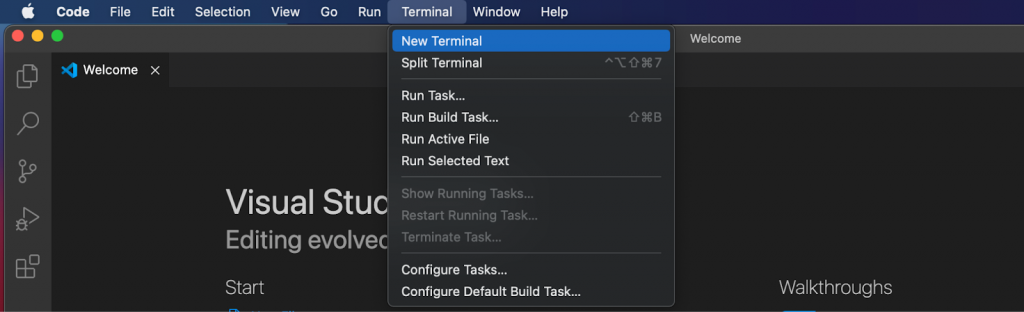
Subsequent up, you could ”cd” into the mission’s root folder and run the next:
python3 -m venv venv
It will arrange a digital surroundings, and it’s best to now discover a folder referred to as ”venv” in your native listing:
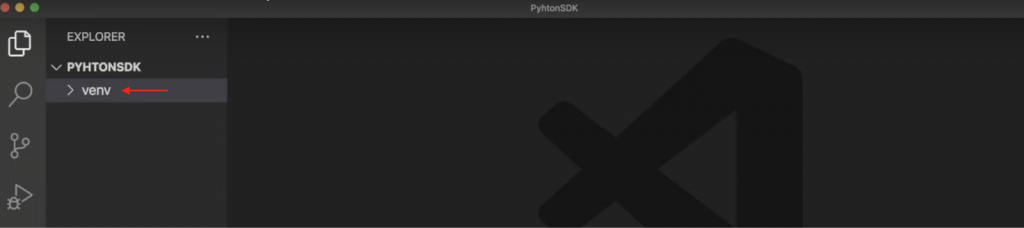
From there, initialize this digital surroundings by inputting the command beneath into the terminal and hitting enter:
supply venv/bin/activate
As soon as the surroundings has been initialized, just be sure you have the newest variations of ”pip” by way of this terminal enter:
pip set up --upgrade pip
Lastly, to conclude the preliminary setup of the barebones state of the mission, you could set up the required dependencies. There are three in complete, and you’ll set up them by working the instructions beneath in consecutive order:
pip set up flask
pip set up flask_cors
pip set up moralis
With the essential template in your mission at hand, the following sub-section breaks down the code you could implement to make the backend software work as supposed!
Software Code
With the mission all arrange, we’ll take this sub-section of the Web3 py tutorial to discover the applying code. As such, create a brand new ”app.py” file within the mission’s root folder. Then, begin by importing the required dependencies on the high:
from flask import Flask from flask import request from moralis import auth from flask_cors import CORS
From there, initialize the app and wrap the app in ”CORS”. To take action, add this snippet of code beneath the imports:
app = Flask(__name__) CORS(app)
Subsequent up, create a brand new variable in your Moralis API key:
api_key = "xxx"
Nonetheless, as you might need figured, you could exchange ”xxx” along with your key. To get it, enroll with Moralis and log in to the admin panel. You may then discover the important thing by navigating to the ”Web3 APIs” tab:
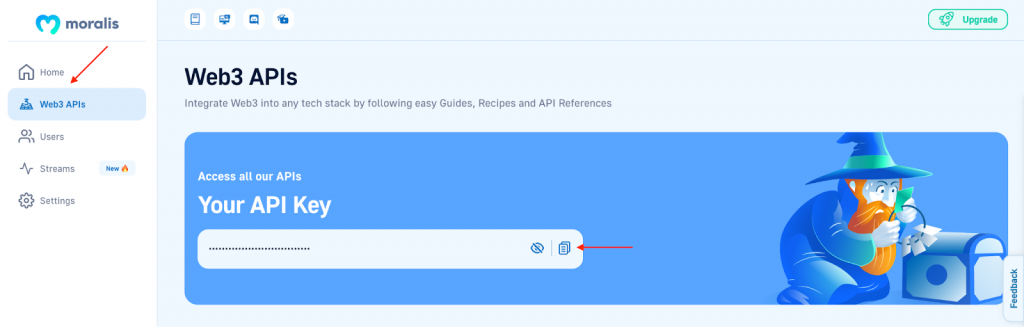
From there, you now must create two routes which might be liable for dealing with the backend software logic!
Route – “/requestChallenge”
This preliminary route is liable for requesting a problem at any time when a consumer needs to authenticate. The central a part of this route is the ”reqChallenge()” operate. Additional, this operate initially fetches the request arguments, units up a brand new ”physique” variable, acquires the outcomes from the ”/requestChallenge” endpoint, and eventually delivers the outcomes to the shopper:
@app.route('/requestChallenge', strategies=["GET"]) def reqChallenge(): args = request.args physique = { "area": "my.dapp", "chainId": args.get("chainId"), "tackle": args.get("tackle"), "assertion": "Please verify login", "uri": "https://my.dapp/", "expirationTime": "2023-01-01T00:00:00.000Z", "notBefore": "2020-01-01T00:00:00.000Z", "assets": ['https://docs.moralis.io/'], "timeout": 30, } outcome = auth.problem.request_challenge_evm( api_key=api_key, physique=physique, ) return outcome
Route – “/verifyChallenge”
The second route is liable for verifying the messages signed by customers. This route comprises the ”verifyChallenge()” operate, which is liable for getting the arguments from the requests, creating new ”physique” variables, fetching the outcomes from Moralis’ Auth API, and returning the outcomes to the purchasers:
@app.route('/verifyChallenge', strategies=["GET"]) def verifyChallenge(): args = request.args physique={ "message": args.get("message"), "signature": args.get("signature"), } outcome = auth.problem.verify_challenge_evm( api_key=api_key, physique=physique ) return outcome
Lastly, after the 2 routes, you could specify the place you need to run the applying. To take action, enter the next:
if __name__ == "__main__": app.run(host="127.0.0.1", port=3000, debug=True)
Nonetheless, that’s it for the applying code! It’s best to now have a file with code just like the one proven beneath:
from flask import Flask from flask import request from moralis import auth from flask_cors import CORS app = Flask(__name__) CORS(app) api_key = "xxx" @app.route('/requestChallenge', strategies=["GET"]) def reqChallenge(): args = request.args physique = { "area": "my.dapp", "chainId": args.get("chainId"), "tackle": args.get("tackle"), "assertion": "Please verify login", "uri": "https://my.dapp/", "expirationTime": "2023-01-01T00:00:00.000Z", "notBefore": "2020-01-01T00:00:00.000Z", "assets": ['https://docs.moralis.io/'], "timeout": 30, } outcome = auth.problem.request_challenge_evm( api_key=api_key, physique=physique, ) return outcome @app.route('/verifyChallenge', strategies=["GET"]) def verifyChallenge(): args = request.args physique={ "message": args.get("message"), "signature": args.get("signature"), } outcome = auth.problem.verify_challenge_evm( api_key=api_key, physique=physique ) return outcome if __name__ == "__main__": app.run(host="127.0.0.1", port=3000, debug=True)
Step 2: Begin the App
With all of the code added to the mission, that concludes the preliminary step of this Web3 py tutorial. Now, on this second step, we’ll present you the right way to run the applying. Operating the app is straightforward, simply open a brand new terminal, enter the next command, and hit enter:
python3 app.py
When you run the command above, it autonomously spins up your software on “localhost 3000” since that is what you laid out in step one. Consequently, you now know the way Web3 py works and may create a backend software dealing with the logic for a Web3 authentication circulation! Nonetheless, allow us to additionally take a more in-depth have a look at the third step of this Web3 py tutorial, the place we’ll illustrate the right way to simply arrange a React software for testing the endpoints and utilizing the Web3 authentication circulation in apply!
Step 3: Set Up the React Software
Now that you’ve got accomplished the preliminary two steps of the Web3 py tutorial and know the right way to create a Web3 backend Python software, allow us to soar straight into the third step. This part will present you the right way to arrange a simple React software for calling the endpoints and implementing the Web3 authentication circulation in apply!
To make this extra accessible, we have now already constructed a React software which you can make the most of. Therefore, you solely want to go to the GitHub repo beneath and clone the mission to your system:
Full Web3 Py Tutorial Documentation – https://github.com/MoralisWeb3/youtube-tutorials/tree/principal/Web3AuthPython
With a neighborhood copy of the repository, it’s best to now have the same file construction in your IDE because the one within the print display screen beneath:
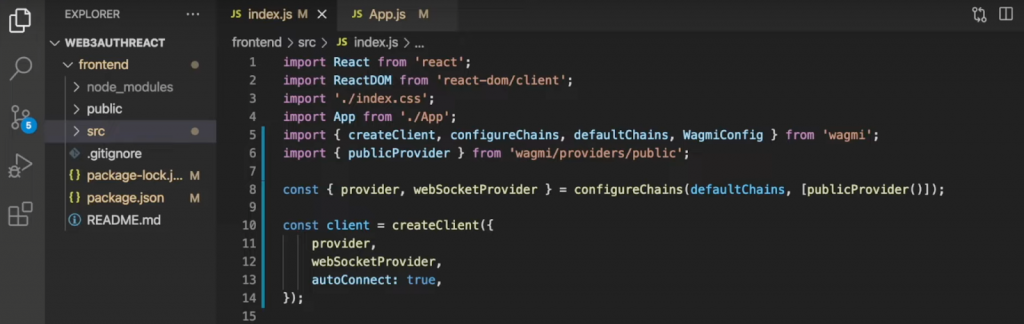
Lastly, all that continues to be from right here is beginning the app. So as to take action, open a terminal and run this command:
npm run begin
That’s it! Congratulations! You will have now accomplished the three steps of this Web3 py tutorial! From right here, it’s best to now be capable of launch the React frontend software and check it out to verify every little thing works as supposed!
If you’re on the lookout for a extra detailed breakdown of the whole course of and frontend code, try the video beneath from the Moralis YouTube channel. On this clip, one in every of Moralis’ software program engineers supplies an much more in depth walkthrough of the whole Web3 py tutorial from begin to end:
Moreover, you may also try the official Web3 Python SDK documentation for extra info on the event package’s capabilities!
Final Web3 Py Tutorial – Abstract
On this Web3 py tutorial, we taught you the right way to create an software permitting customers to register with their MetaMask wallets. The appliance consisted of two core parts: a backend Python software and a frontend React software. What’s extra, due to Moralis’ Python SDK, you had been capable of create this app in solely three steps:
- Create the Python Flask Software
- Begin the App
- Set Up the React Software
You probably have adopted alongside this far, you now know the right way to implement Web3 performance into Python purposes!
If this tutorial for py improvement was useful, take into account trying out further Moralis articles right here on the Web3 weblog. For example, study to get NFT collections utilizing Python or arrange automated Web3 notification emails! What’s extra, don’t forget to enroll with Moralis in case you are trying to turn out to be a Web3 developer. Creating an account is free and solely takes a few seconds, so you don’t have anything to lose!