Right now’s tutorial demonstrates the best way to get the ERC20 token steadiness of an handle with the Moralis Token API. Due to the accessibility of this instrument, all you want is a single API name to the getWalletTokenBalances()
endpoint. Right here’s an instance of what it may possibly appear to be:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); const runApp = async () => { await Moralis.begin({ apiKey: "YOUR_API_KEY", // ...and another configuration }); const handle = "0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d"; const chain = EvmChain.ETHEREUM; const response = await Moralis.EvmApi.token.getWalletTokenBalances({ handle, chain, }); console.log(response.toJSON()); }; runApp();
All you need to do is change YOUR_API_KEY
together with your Moralis API key, configure the handle
and chain
parameters to suit your request, and run the code. In return, you’ll get an array of tokens containing the steadiness for every:
[ { "token_address": "0x3c978fc9a42c80a127863d786d8883614b01b3cd", "symbol": "USDT", "name": "USDTOKEN", "logo": null, "thumbnail": null, "decimals": 18, "balance": "10000000000000000000000", "possible_spam": true }, { "token_address": "0xdac17f958d2ee523a2206206994597c13d831ec7", "symbol": "USDT", "name": "Tether USD", "logo": "https://cdn.moralis.io/eth/0xdac17f958d2ee523a2206206994597c13d831ec7.png", "thumbnail": "https://cdn.moralis.io/eth/0xdac17f958d2ee523a2206206994597c13d831ec7_thumb.png", "decimals": 6, "balance": "102847", "possible_spam": false }, //... ]
That’s it; when working with Moralis, it’s simple to get the ERC20 token steadiness of an handle. From right here, now you can use this knowledge and effortlessly combine it into your Web3 initiatives!
When you’d wish to be taught extra about how this works, be a part of us on this article or try the official documentation on the best way to get ERC20 token steadiness by a pockets.
Additionally, bear in mind to enroll with Moralis, as you’ll want an account to observe alongside on this tutorial. You possibly can register fully free of charge, and also you’ll acquire rapid entry to the business’s main Web3 APIs!
Overview
A vital side of most blockchain functions – together with wallets, decentralized exchanges (DEXs), portfolio trackers, and Web3 analytics instruments – are ERC20 token balances. Consequently, it doesn’t matter what decentralized utility (dapp) you’re constructing, you’ll doubtless want the power to effortlessly get the ERC20 token steadiness of an handle. Nevertheless, querying and processing this knowledge with out correct growth instruments is a tedious and time-consuming job. Thankfully, now you can keep away from the difficult elements by leveraging the Moralis Token API to get the ERC20 token steadiness of an handle very quickly!
In at the moment’s article, we’ll begin by going again to fundamentals and briefly look at what a token steadiness is. From there, we’re additionally going to discover ERC20, as that is the most well-liked customary for fungible tokens. Subsequent, we’ll leap straight into the tutorial and present you the best way to seamlessly get the ERC20 token steadiness of an handle utilizing the Moralis Token API. To high issues off, we’ll additionally present you the best way to use the Token API to get the spender allowance of an ERC20 token!
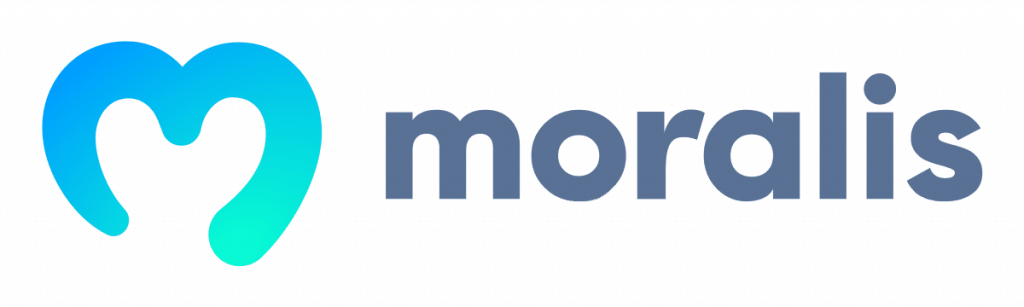
When you already know what a token steadiness is and what the ERC20 token customary entails, be at liberty to skip straight into the ”Tutorial: Learn how to Get the ERC20 Token Steadiness of an Deal with” part!
Additionally, in case you’re critical about constructing Web3 initiatives, you’ll doubtless wish to try another instruments Moralis gives in addition to the Token API. For example, two different wonderful interfaces are Moralis’ free NFT API and the free crypto Value API. With these instruments, you may seamlessly construct Web3 initiatives in a heartbeat!
So, if you would like entry to the business’s main Web3 APIs, bear in mind to enroll with Moralis so you can begin leveraging the true energy of blockchain know-how at the moment!
What’s a Token Steadiness?
A token steadiness refers back to the quantity of a selected cryptocurrency an account or pockets holds. Within the crypto world, there are two main fungible token sorts: native and non-native tokens. Let’s have a look at every somewhat bit deeper:
- Native Tokens: A local token is a blockchain community’s base cryptocurrency. All unbiased blockchains have their very own native token. And so they have loads of utility inside their respective ecosystems. For example, a local token is usually used to reward validators/miners and pay for transaction charges. A outstanding instance is ETH, which is the native token of the Ethereum community.
- Non-Native Tokens: Non-native tokens are created on high of an current community – similar to Ethereum – utilizing good contracts. These tokens are sometimes created by a Web3 mission and are solely used inside its personal borders. Examples of non-native tokens embody DAI, USDT, and USDC.
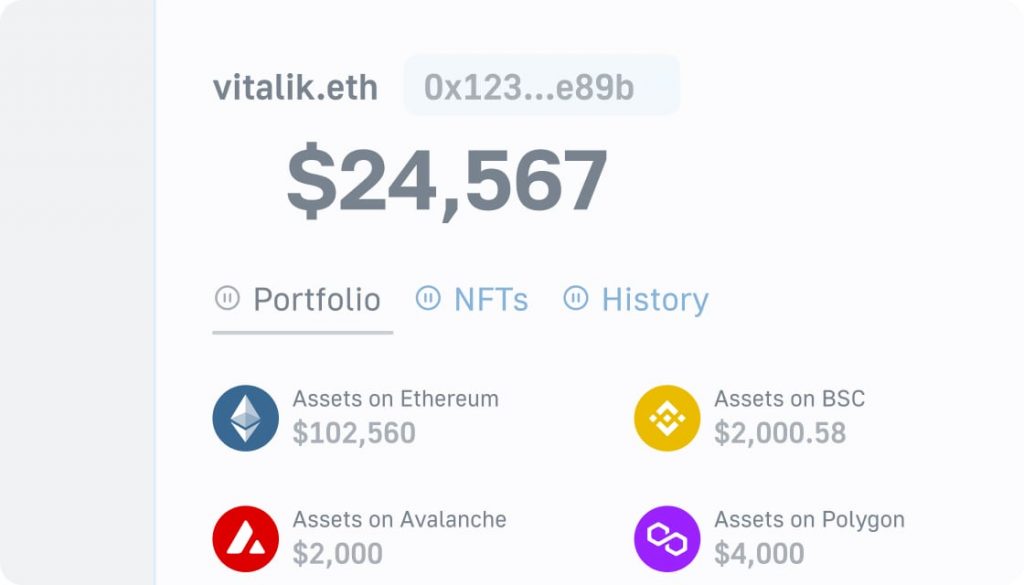
So, when speaking a few token steadiness, we sometimes discuss with the quantity of a selected native or non-native token an account or pockets holds. Furthermore, regardless that there are numerous forms of fungible tokens, they largely observe the ERC20 token customary. However what precisely does this imply? And the way does ERC20 work?
What’s ERC20?
ERC20, or Ethereum Request for Remark 20, is a technical customary for good contracts on the Ethereum blockchain and different EVM-compatible networks. This customary defines a algorithm, features, and occasions {that a} token should implement for it to be thought-about ERC-20 compliant.
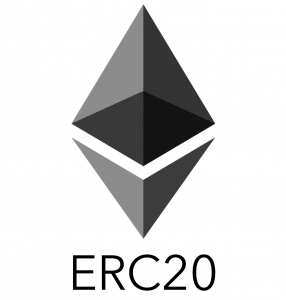
The features and occasions outlined within the ERC20 token customary present a typical interface for all tokens to allow them to be simply accessed, acknowledged, and used. For example, one of many features is balanceOf()
, guaranteeing that it’s attainable to seamlessly get a consumer’s steadiness of any ERC20 token.
However, this customary interface ensures a unified ecosystem the place all tokens are suitable with each other, together with decentralized functions (dapps) and different Web3 initiatives. Some outstanding examples of ERC20 tokens embody ETH, USDC, USDT, SHIB, and lots of others!
Additionally, whereas ERC20 is the most well-liked and well-used token customary, it’s removed from the one one. Two further requirements are ERC721 and ERC1155, that are used to control NFTs on the Ethereum blockchain. When you’d wish to be taught extra about these, try our article on ERC721 vs. ERC1155!
However, now that you’ve a greater understanding of what ERC20 entails, let’s leap straight into the tutorial and present you the best way to get a pockets’s ERC20 token steadiness!
Tutorial: Learn how to Get the ERC20 Token Steadiness of an Deal with
The most effective and most simple technique to get the ERC20 token steadiness of a consumer is to leverage the Moralis Token API. This API offers real-time ERC20 token knowledge, permitting you to fetch costs, transfers, and, in fact, balances with a single line of code!
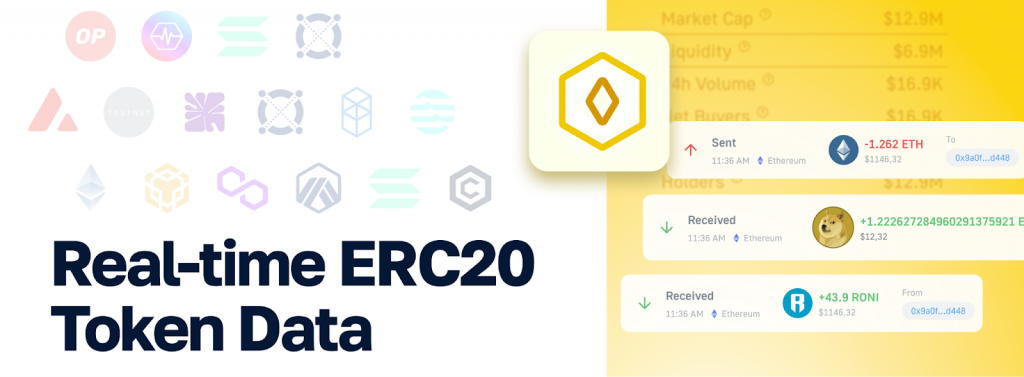
The API can be cross-chain suitable, supporting ten-plus EVM chains, together with Ethereum, BNB Sensible Chain, Polygon, and lots of others. As such, with Moralis, it has by no means been simpler to construct crypto wallets, portfolio trackers, or Web3 token analytics instruments!
However, to focus on the accessibility of this instrument, we’ll take the next sections to indicate you the best way to get the ERC20 token steadiness of a consumer in three simple steps:
- Get an API Key
- Write a Script
- Run the Code
However earlier than we leap into step one of the tutorial, you’ll need to maintain just a few conditions!
Conditions
On this tutorial, we’ll present the best way to get the ERC20 token steadiness of a consumer with JavaScript. Nevertheless, you may also use TypeScript and Python in case you desire. However if that is so, be aware that the steps outlined on this tutorial would possibly differ barely.
However, earlier than you get going, you’ll must have the next prepared:
From right here, you must set up the Moralis SDK. To take action, arrange a brand new mission in your built-in growth atmosphere (IDE) and run the next terminal command in your mission’s root folder:
npm set up moralis @moralisweb3/common-evm-utils
That’s it; you’re now prepared to leap into step one of this tutorial on the best way to get the ERC20 token steadiness of a consumer!
Step 1: Get an API Key
You’ll initially want a Moralis API key, which is required for making calls to the Token API. Thankfully, you will get your API key free of charge. All you need to do is enroll with Moralis by clicking on the ”Begin for Free” button on the high proper of the Moralis homepage:
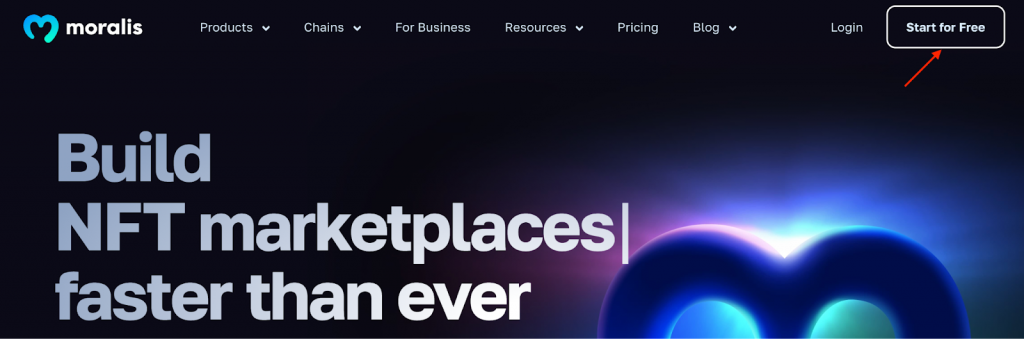
When you’re accomplished establishing an account and your first mission, you’ll find the important thing by navigating to the ”Settings” tab. From there, you may merely scroll down and duplicate your Moralis API key:
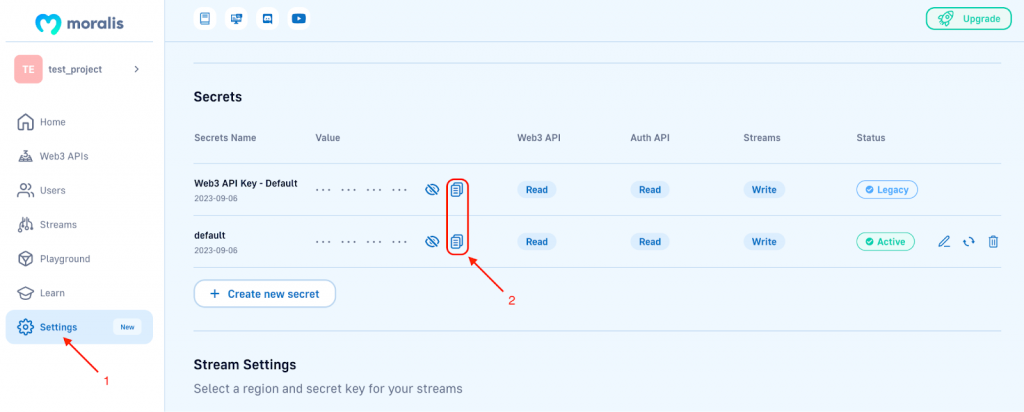
Save the important thing, as you’ll want it within the subsequent step!
Step 2: Write a Script
With a Moralis API key at hand, you’re prepared to start out coding. As such, arrange a brand new ”index.js” file in your mission’s root folder and add the next code:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); const runApp = async () => { await Moralis.begin({ apiKey: "YOUR_API_KEY", // ...and another configuration }); const handle = "0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d"; const chain = EvmChain.ETHEREUM; const response = await Moralis.EvmApi.token.getWalletTokenBalances({ handle, chain, }); console.log(response.toJSON()); }; runApp();
That is all of the code you must get the ERC20 token steadiness of a consumer. Nevertheless, you must make just a few minor configurations earlier than you may run the script.
Initially, change YOUR_API_KEY
with the important thing you copied within the earlier step:
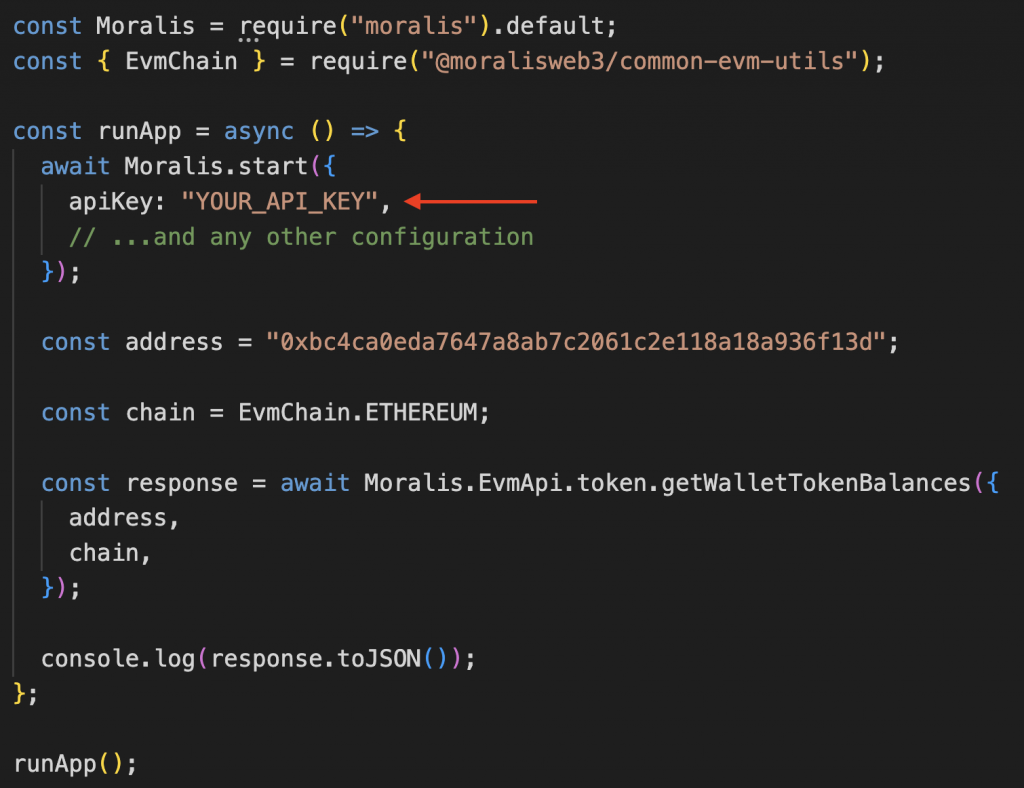
Subsequent, you must configure the handle
and chain
parameters to suit your request. For the handle
const, add the pockets handle from which you wish to get the ERC20 token steadiness. And for chain
, you may change the community in case you want to question a blockchain aside from Ethereum:
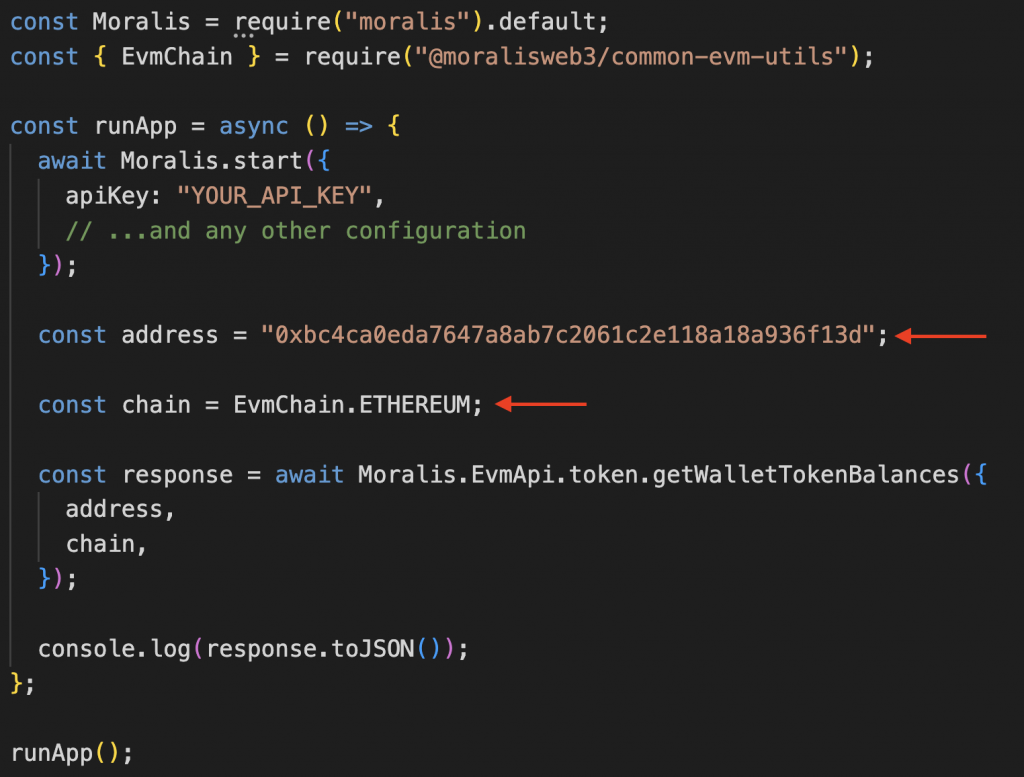
From there, we then cross the parameters when calling the getWalletTokenBalances()
endpoint:

That is it; that’s all of the code you must get the ERC20 token steadiness of a consumer. All that continues to be from right here is operating the script!
Step 3: Run the Code
To run the script, merely open a terminal, cd
into the mission’s root folder, and execute the next command:
node index.js
In return, you’ll get a response containing an array of ERC20 tokens together with the steadiness for every cryptocurrency. Right here’s an instance of what it’d appear to be:
[ { "token_address": "0x3c978fc9a42c80a127863d786d8883614b01b3cd", "symbol": "USDT", "name": "USDTOKEN", "logo": null, "thumbnail": null, "decimals": 18, "balance": "10000000000000000000000", "possible_spam": true }, { "token_address": "0xdac17f958d2ee523a2206206994597c13d831ec7", "symbol": "USDT", "name": "Tether USD", "logo": "https://cdn.moralis.io/eth/0xdac17f958d2ee523a2206206994597c13d831ec7.png", "thumbnail": "https://cdn.moralis.io/eth/0xdac17f958d2ee523a2206206994597c13d831ec7_thumb.png", "decimals": 6, "balance": "102847", "possible_spam": false }, //... ]
From right here, now you can effortlessly combine the ERC20 token steadiness knowledge into your initiatives!
Learn how to Get Spender Allowance of an ERC20 Token
Along with getting the ERC20 token steadiness of a consumer, the Moralis Token API additionally permits you to question the spender allowance of an ERC20 token. However what precisely is the spender allowance?
ERC20 token allowance refers back to the quantity {that a} spender is allowed to withdraw on behalf of the proprietor. That is widespread when interacting with dapps like Uniswap, giving the platform the suitable to switch the tokens you maintain in your pockets.
So, how will you get the spender allowance of an ERC20 token?
Nicely, due to the accessibility of the Moralis Token API, you may observe the identical steps from the ”Tutorial: Learn how to Get the ERC20 Token Steadiness…” part. The one distinction is that you must name the getTokenAllowance()
endpoint as an alternative of getWalletTokenBalances()
.
As such, if you wish to get the spender allowance of an ERC20 token, merely change the contents in your ”index.js” file with the next code:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); const runApp = async () => { await Moralis.begin({ apiKey: "YOUR_API_KEY", // ...and another configuration }); const chain = EvmChain.ETHEREUM; const handle = "0x514910771AF9Ca656af840dff83E8264EcF986CA"; const ownerAddress = "0x7c470D1633711E4b77c8397EBd1dF4095A9e9E02"; const spenderAddress = "0xed33259a056f4fb449ffb7b7e2ecb43a9b5685bf"; const response = await Moralis.EvmApi.token.getTokenAllowance({ handle, chain, ownerAddress, spenderAddress, }); console.log(response.toJSON()); }; runApp();
From right here, you’ll then want so as to add your API key by changing YOUR_API_KEY
:
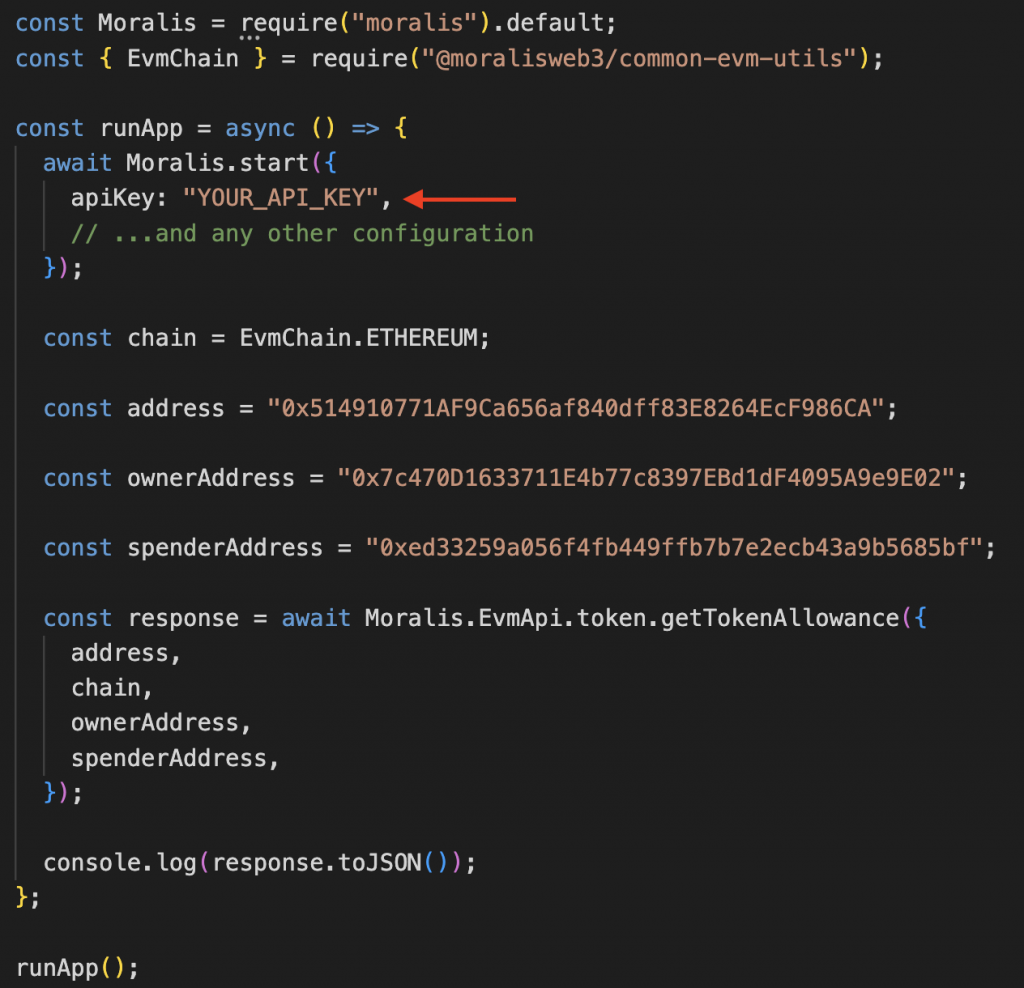
Subsequent, you have to configure the parameters, and as you’ll shortly discover, there are just a few extra this time. For the handle
parameter, you must add the token handle. For ownerAddress
, add the handle of the token proprietor. And for spenderAddress
, add the handle of the token spender:
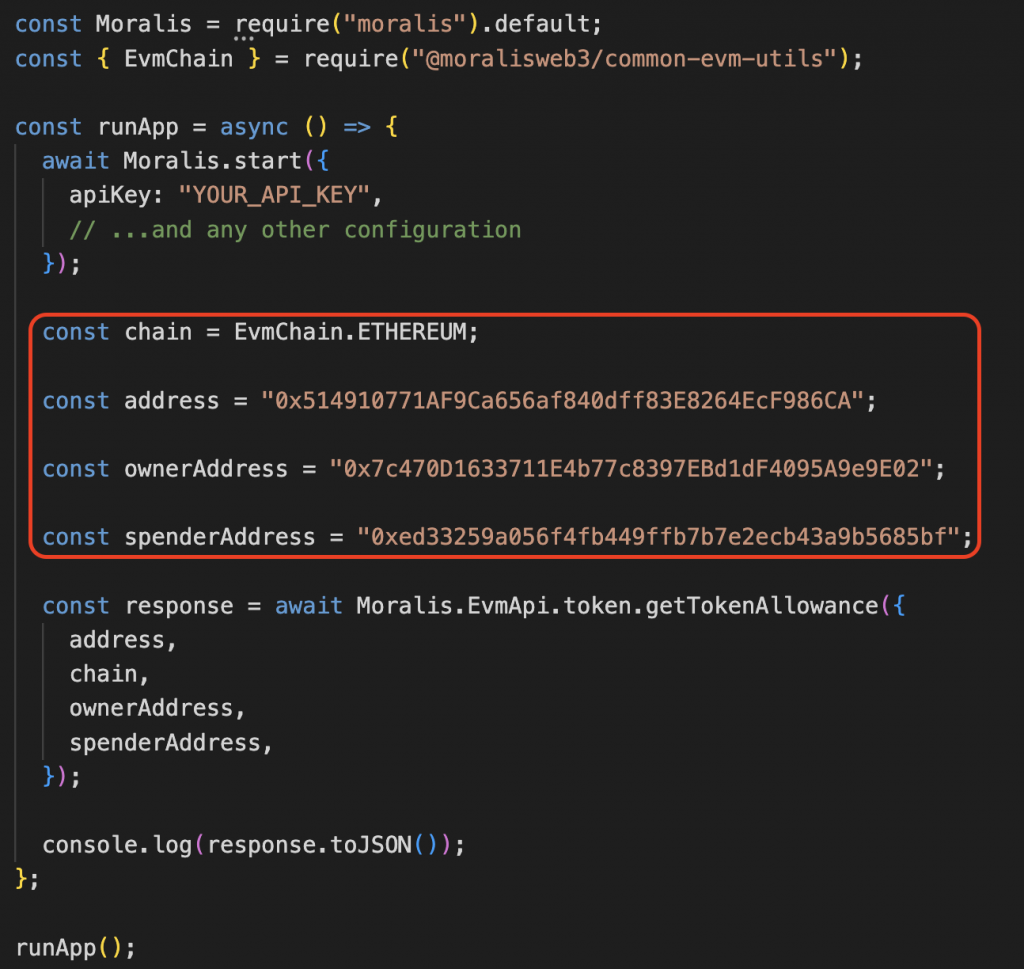
We then cross these parameters when calling the getTokenAllowance()
endpoint:
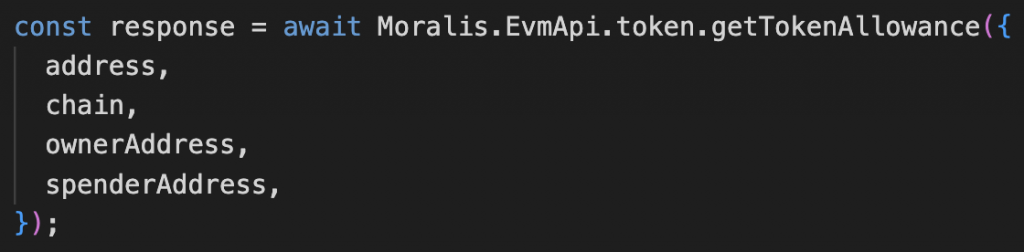
When you run the code, you’ll get a response wanting one thing like this:
{ "allowance": "0" }
And that’s it! That is how simple it’s to get the spender allowance of an ERC20 token when working with Moralis!
For a extra detailed breakdown of how this works, try the get spender allowance of an ERC20 token documentation!
Abstract: Learn how to Get the Steadiness of ERC20 Tokens
In at the moment’s article, we launched you to ERC20 token balances. In doing so, we defined what they’re and how one can get the ERC20 token steadiness of a consumer with the Moralis Value API in three steps:
- Get an API Key
- Write a Script
- Run the Code
If in case you have adopted alongside this far, you now know the best way to get the ERC20 token steadiness of any handle. Now you can use this newly acquired ability to start out constructing your first Web3 mission!
If that is your ambition, then it is best to know that the Token API works like a dream along with our suite of further crypto APIs. You possibly can, as an illustration, mix the Token API with the Moralis NFT API to combine NFT knowledge into your initiatives. When you’d wish to be taught extra about this, try our articles on the best way to get NFT ERC721 on-chain metadata or the best way to get all NFT tokens owned by a consumer handle.
Additionally, in case you favored this tutorial, take into account trying out further content material right here on the Web3 weblog. For instance, dive under consideration abstraction, discover the business’s main NFT picture API, or learn to write a sensible contract in Solidity!
Lastly, don’t neglect to enroll with Moralis if you wish to entry the business’s main Web3 APIs. Creating an account is free, and as a consumer of Moralis, you’ll be capable to construct initiatives quicker and smarter!